Da estilo a tus textos con NSAttributedString
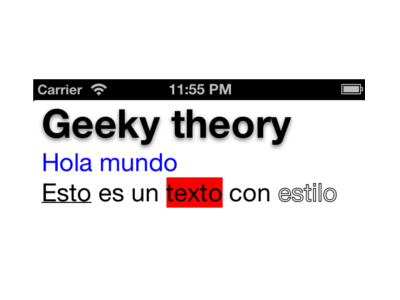
Seguramente en varias ocasiones, en nuestra aplicación, nos hemos encontrado con la necesidad de incluir textos en los cuales queremos resaltar ciertas partes mostrandolas con otro tipo de fuente, espaciado, subrayado o color. Por ello, para darle estilo a las cadenas de texto, en los frameworks Mac OS X Application Kit y iOS UIKit, disponemos de la clase NSAttributedString. NSAttributedString y NSMutableAttributedString son clases que manejan cadenas de caracteres y una serie de atributos gráficos asociados, que se aplican a caracteres individuales o rangos de caracteres en la cadena. Estas clases, pensadas para manejar los atributos gráficos de los textos que encontramos en archivos RTF, RTFD y HTML, se encuentran disponibles desde la version 10.0 de Mac OS X. Sin embargo, en iOS, aunque se encuentran disponibles desde el SDK de iOS 3.2, no ha sido hasta el SDK de iOS 6 cuando se ha incorporado la posibilidad de utilizar estas clases dentro de los controles de interfaz de usuario como UILabel, UIButton, UITextView. En Mac OS X disponemos de los siguientes atributos standar, más otros referidos al manejo de documentos RTF, RTFD y HTML:
- NSFontAttributeName: para personalizar el tipo de fuente del texto.
- NSParagraphStyleAttributeName: para aplicar un estilo personalizado a un párrafo.
- NSForegroundColorAttributeName: para modificar el color de la fuente para el texto.
- NSUnderlineStyleAttributeName: para configurar el subrayado para el texto. (NSUnderlineStyleNone, NSUnderlineStyleSingle, NSUnderlineStyleThick, NSUnderlineStyleDouble)
- NSSuperscriptAttributeName
- NSBackgroundColorAttributeName: para el color de fondo del texto.
- NSAttachmentAttributeName
- NSLigatureAttributeName
- NSBaselineOffsetAttributeName
- NSKernAttributeName
- NSLinkAttributeName
- NSStrokeWidthAttributeName: para el grosor del trazo para la fuente seleccionada. Se aplica un porcentaje sobre el tamaño que ya tuviera seleccionado la fuente de texto mediante NSFontAttributeName.
- NSStrokeColorAttributeName: el color del trazo para la fuente de nuestro texto. Por defecto hace uso del valor introducido en NSForegroundColorAttributeName.
- NSUnderlineColorAttributeName
- NSStrikethroughStyleAttributeName: para aplicar tachado al texto.
- NSStrikethroughColorAttributeName: para definir el color del tachado.
- NSShadowAttributeName: para definir una sombra configurable para el texto con la clase NSShadow (introducida en iOS 6) donde es posible configurar el desplazamiento de la sombra, color, y si produce difuminado en la misma.
- NSObliquenessAttributeName
- NSExpansionAttributeName
- NSCursorAttributeName
- NSToolTipAttributeName
- NSMarkedClauseSegmentAttributeName
- NSWritingDirectionAttributeName
- NSVerticalGlyphFormAttributeName: para configurar texto vertical (1) o texto horizontal (0).
- NSTextAlternativesAttributeName
No obstante, en iOS solo disponemos de:
- NSFontAttributeName
- NSParagraphStyleAttributeName
- NSForegroundColorAttributeName
- NSBackgroundColorAttributeName
- NSLigatureAttributeName
- NSKernAttributeName
- NSStrikethroughStyleAttributeName
- NSUnderlineStyleAttributeName
- NSStrokeColorAttributeName
- NSStrokeWidthAttributeName
- NSShadowAttributeName
- NSVerticalGlyphFormAttributeName
Vamos ahora con un ejemplo para iOS en el que mostraremos un texto con distintos atributos dentro de un UITextView. Para ello abrimos Xcode y creamos un nuevo proyecto iOS Single View Application. Nos vamos a nuestro ViewController y en el metodo que viewDidLoad escribimos el siguiente código: [super viewDidLoad]; NSString *str = @"Geeky theoryrHola mundorEsto es un texto con estilo"; // Usemos NSMutableAttributedString para poder modificar la cadena una vez creada NSMutableAttributedString *stylishStr = [[NSMutableAttributedString alloc] initWithString:str]; // Definimos la fuente del texto [stylishStr addAttribute:NSFontAttributeName value:[UIFont systemFontOfSize:24.0f] range:NSMakeRange(0, [str length])]; // Colocamos el titulo con otro tamaño y en negrita [stylishStr addAttribute:NSFontAttributeName value:[UIFont boldSystemFontOfSize:38.0f] range:NSMakeRange(0,12)]; // También vamos a darle sombra al título NSShadow *shadow = [[NSShadow alloc] init]; [shadow setShadowBlurRadius:4.0]; [shadow setShadowColor:[UIColor grayColor]]; [shadow setShadowOffset:CGSizeMake(0, 2)]; // Cambiamos el atributo [stylishStr addAttribute:NSShadowAttributeName value:shadow range:NSMakeRange(0, 12)]; // Probemos con distintos colores [stylishStr addAttribute:NSForegroundColorAttributeName value:[UIColor blueColor] range:NSMakeRange(13,10)]; // Subrayado [stylishStr addAttribute:NSUnderlineStyleAttributeName value:[NSNumber numberWithInt:NSUnderlineStyleSingle] range:NSMakeRange(24, 4)]; // Color de fondo [stylishStr addAttribute:NSBackgroundColorAttributeName value:[UIColor redColor] range:NSMakeRange(35, 5)]; // O el grosor del trazo [stylishStr addAttribute:NSStrokeWidthAttributeName value:[NSNumber numberWithFloat:3.0] range:NSMakeRange(45, 6)]; // Por último mostramos todo esto en un UITextView UITextView * textView = [[UITextView alloc] initWithFrame:self.view.frame]; [textView setAttributedText:stylishStr]; self.view = textView;
Compilamos y ejecutamos obteniendo el siguiente resultado:
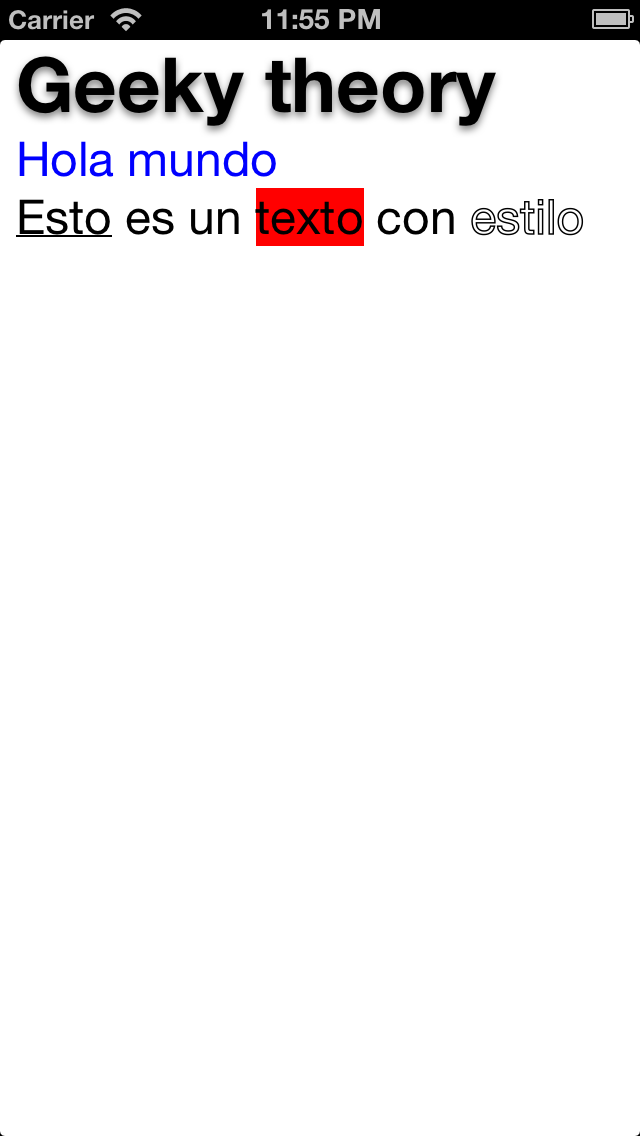
Por último, recordar que este código solo sera compatible con iOS 6 o superior.