Curso Principios SOLID - 4. Principio de Segregación de Interfaces
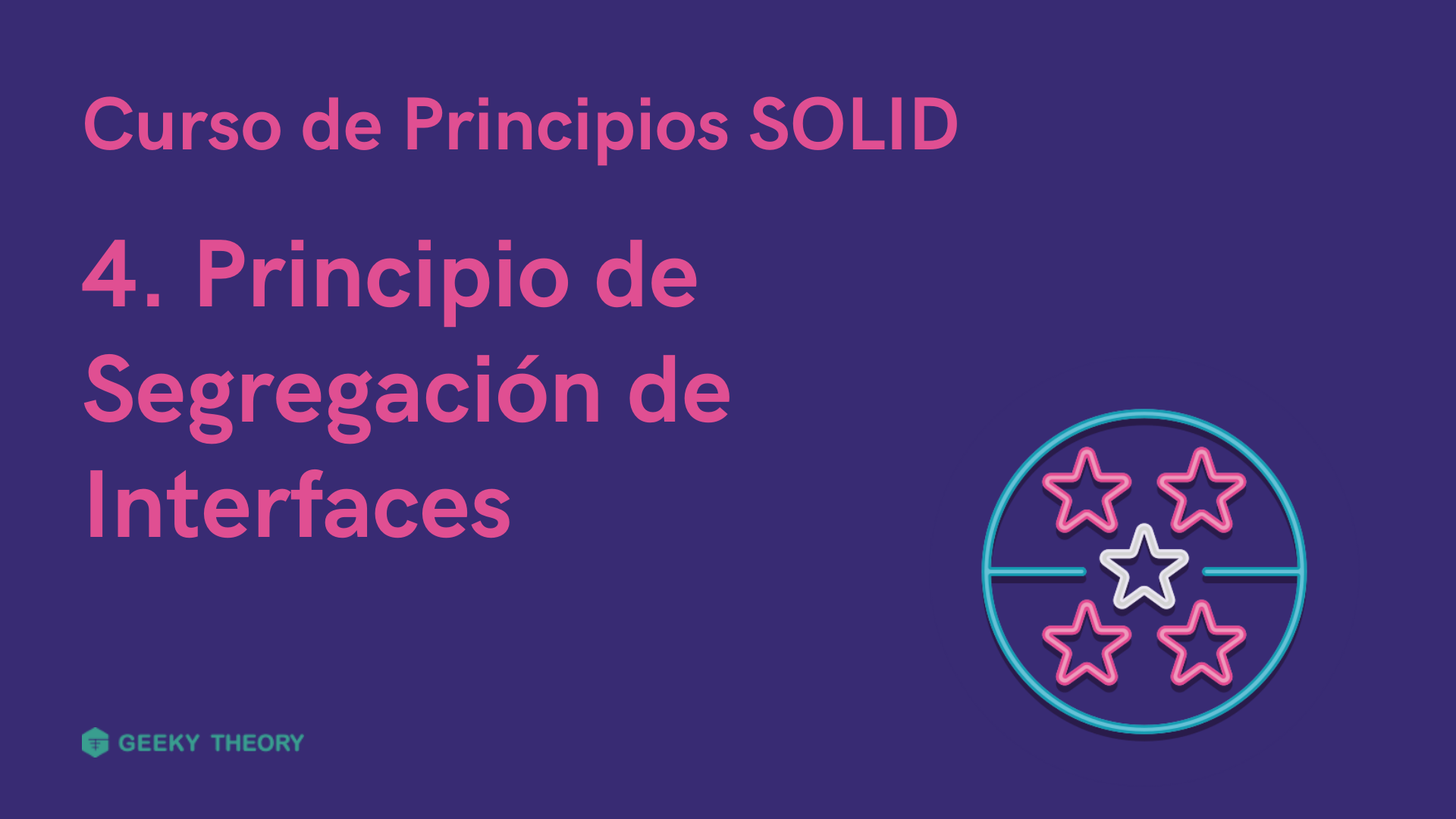
La I de SOLID representa el Principio de Segregación de Interfaces (ISP), que establece que ninguna clase debería depender de métodos que no utiliza.
¿Por qué debemos seguir este principio? Evita que haya métodos innecesarios en determinadas clases.
Ejemplo práctico
<?php
interface WorkerInterface
{
public function work();
public function rest();
public function eat();
}
class Mechanic implements WorkerInterface
{
public function work()
{
echo "Working on mechanical things...";
}
public function rest()
{
echo "Resting...";
}
public function eat()
{
echo "Eating...";
}
}
class Robot implements WorkerInterface
{
public function work()
{
echo "Working on robotic things...";
}
public function rest()
{
// A robot does not rest
}
public function eat()
{
// A robot does not eat
}
}
A continuación vamos a refactorizar el código para no violar el Principio de Segregación de Interfaces:
<?php
interface WorkableInterface
{
public function work();
}
interface RestableInterface
{
public function rest();
}
interface FeedableInterface
{
public function eat();
}
class Mechanic implements WorkableInterface, RestableInterface, FeedableInterface
{
public function work()
{
echo "Working on mechanical things...";
}
public function rest()
{
echo "Resting...";
}
public function eat()
{
echo "Eating...";
}
}
class Robot implements WorkableInterface
{
public function work()
{
echo "Working on robotic things...";
}
}
class Programmer implements WorkableInterface, FeedableInterface
{
public function work()
{
echo "Coding...";
}
public function eat()
{
echo "Eating pizza...";
}
}
Vamos a hacer otro ejemplo con impresoras:
<?php
interface DeviceInterface
{
public function print();
public function fax();
public function scan();
}
class AllInOnePrinter implements DeviceInterface
{
public function print()
{
echo "Printing...";
}
public function fax()
{
echo "Sending fax...";
}
public function scan()
{
echo "Scanning...";
}
}
class CheapPrinter implements DeviceInterface
{
public function print()
{
echo "Printing";
}
public function fax()
{
throw new Exception("Not supported");
}
public function scan()
{
throw new Exception("Not supported");
}
}
Tras aplicar el Principio de Segregación de Interfaces, el código sería el siguiente:
<?php
interface PrinterInterface
{
public function print();
}
interface FaxInterface
{
public function fax();
}
interface ScanInterface
{
public function scan();
}
class AllInOnePrinter implements PrinterInterface, FaxInterface, ScanInterface
{
public function print()
{
echo "Printing...";
}
public function fax()
{
echo "Sending fax...";
}
public function scan()
{
echo "Scanning...";
}
}
class CheapPrinter implements PrinterInterface
{
public function print()
{
echo "Printing";
}
}